C++rsa加密算法代码
作者:野牛程序员:2023-07-05 08:41:44 C++阅读 2784
以下是一个简单的示例代码,演示了如何使用C++实现RSA加密算法:
#include <iostream> #include <cmath> #include <vector> using namespace std; // 求最大公约数 int gcd(int a, int b) { if (b == 0) return a; else return gcd(b, a % b); } // 判断是否为素数 bool isPrime(int n) { if (n <= 1) return false; int sqrtN = sqrt(n); for (int i = 2; i <= sqrtN; i++) { if (n % i == 0) return false; } return true; } // 生成RSA公钥和私钥 void generateRSAKeys(int p, int q, int& publicKey, int& privateKey) { // 检查输入的两个数是否为素数 if (!isPrime(p) || !isPrime(q)) { cout << "p和q必须是素数" << endl; return; } // 计算n和phi(n) int n = p * q; int phiN = (p - 1) * (q - 1); // 选择公钥e(1 < e < phiN):e与phiN互质 for (int e = 2; e < phiN; e++) { if (gcd(e, phiN) == 1) { publicKey = e; break; } } // 计算私钥d:(d * e) mod phiN = 1 int d; for (d = 2; d < phiN; d++) { if ((d * publicKey) % phiN == 1) { privateKey = d; break; } } } // 加密 int encrypt(int plaintext, int publicKey, int n) { int ciphertext = pow(plaintext, publicKey); ciphertext = fmod(ciphertext, n); return ciphertext; } // 解密 int decrypt(int ciphertext, int privateKey, int n) { int plaintext = pow(ciphertext, privateKey); plaintext = fmod(plaintext, n); return plaintext; } int main() { int p, q; // 两个素数 int publicKey, privateKey; // 公钥和私钥 int plaintext; // 明文 int ciphertext; // 密文 // 输入素数p和q cout << "输入两个素数p和q:" << endl; cin >> p >> q; // 生成RSA公钥和私钥 generateRSAKeys(p, q, publicKey, privateKey); // 输出公钥和私钥 cout << "公钥为:" << publicKey << endl; cout << "私钥为:" << privateKey << endl; // 输入明文 cout << "输入要加密的明文:" << endl; cin >> plaintext; // 加密 ciphertext = encrypt(plaintext, publicKey, p * q); cout << "加密后的密文为:" << ciphertext << endl; // 解密 plaintext = decrypt(ciphertext, privateKey, p * q); cout << "解密后的明文为:" << plaintext << endl; return 0; }
请注意,这只是一个简单的示例,用于演示RSA加密算法的基本原理。在实际应用中,需要考虑更多的安全性和性能方面的细节。此外,还应该处理更大的整数,以便支持更安全的密钥长度。在实际使用中,建议使用经过验证和已经实现的加密库来执行RSA加密操作。
野牛程序员教少儿编程与信息学奥赛-微信|电话:15892516892
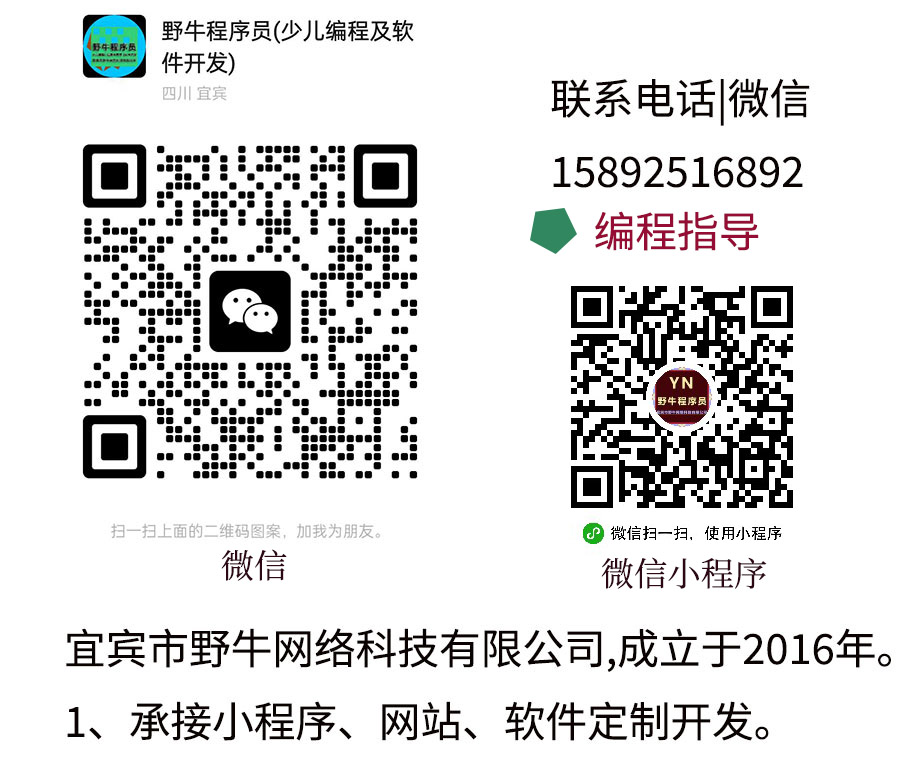