算法中的暴力法指的是什么?
作者:野牛程序员:2023-10-31 14:40:15算法阅读 2687
枚举所有情况,然后判断每一种情况是否合法的做法是非常朴素的。因此一般把不使用优化算法、直接用朴素算法来解决问题的做法称为暴力法。
暴力法是一种朴素的问题解决方法,通常它会枚举所有可能的情况,然后逐一检查每种情况是否符合问题的要求。虽然这种方法通常不够高效,但在一些情况下,它是解决问题的有效方式,特别是在问题规模较小的情况下,或者作为问题的暴力搜索的初始步骤。
以下是一个使用C++的示例,演示如何使用暴力法来解决一个经典问题:寻找给定数组中的两个元素,它们的和等于目标值。
#include <iostream> #include <vector> using namespace std; vector<int> findTwoSum(vector<int> nums, int target) { vector<int> result; for (int i = 0; i < nums.size(); i++) { for (int j = i + 1; j < nums.size(); j++) { if (nums[i] + nums[j] == target) { result.push_back(i); result.push_back(j); return result; } } } return result; } int main() { vector<int> nums = {2, 7, 11, 15}; int target = 9; vector<int> indices = findTwoSum(nums, target); if (indices.size() == 2) { cout << "目标值 " << target << " 的两个元素的索引是: " << indices[0] << " 和 " << indices[1] << endl; } else { cout << "未找到符合条件的两个元素。" << endl; } return 0; }
在上述示例中,使用两个嵌套循环遍历数组中的所有可能组合,并检查它们的和是否等于目标值。如果找到符合条件的组合,就返回它们的索引。这是一个典型的暴力法示例,因为它没有使用任何优化算法,而是简单地考虑了所有可能的情况。
方法二:
#include <iostream> using namespace std; int* findTwoSum(int* nums, int length, int target) { int* result = new int[2]; for (int i = 0; i < length; i++) { for (int j = i + 1; j < length; j++) { if (nums[i] + nums[j] == target) { result[0] = i; result[1] = j; return result; } } } return nullptr; } int main() { int nums[] = {2, 7, 11, 15}; int target = 9; int* indices = findTwoSum(nums, 4, target); if (indices) { cout << "目标值 " << target << " 的两个元素的索引是: " << indices[0] << " 和 " << indices[1] << endl; delete[] indices; } else { cout << "未找到符合条件的两个元素。" << endl; } return 0; }
方法三:
#include <iostream> using namespace std; void findTwoSum(const int nums[], int length, int target, int result[]) { for (int i = 0; i < length; i++) { for (int j = i + 1; j < length; j++) { if (nums[i] + nums[j] == target) { result[0] = i; result[1] = j; return; } } } result[0] = -1; result[1] = -1; } int main() { int nums[] = {2, 7, 11, 15}; int target = 9; int indices[2]; findTwoSum(nums, 4, target, indices); if (indices[0] != -1 && indices[1] != -1) { cout << "目标值 " << target << " 的两个元素的索引是: " << indices[0] << " 和 " << indices[1] << endl; } else { cout << "未找到符合条件的两个元素。" << endl; } return 0; }
野牛程序员教少儿编程与信息学奥赛-微信|电话:15892516892
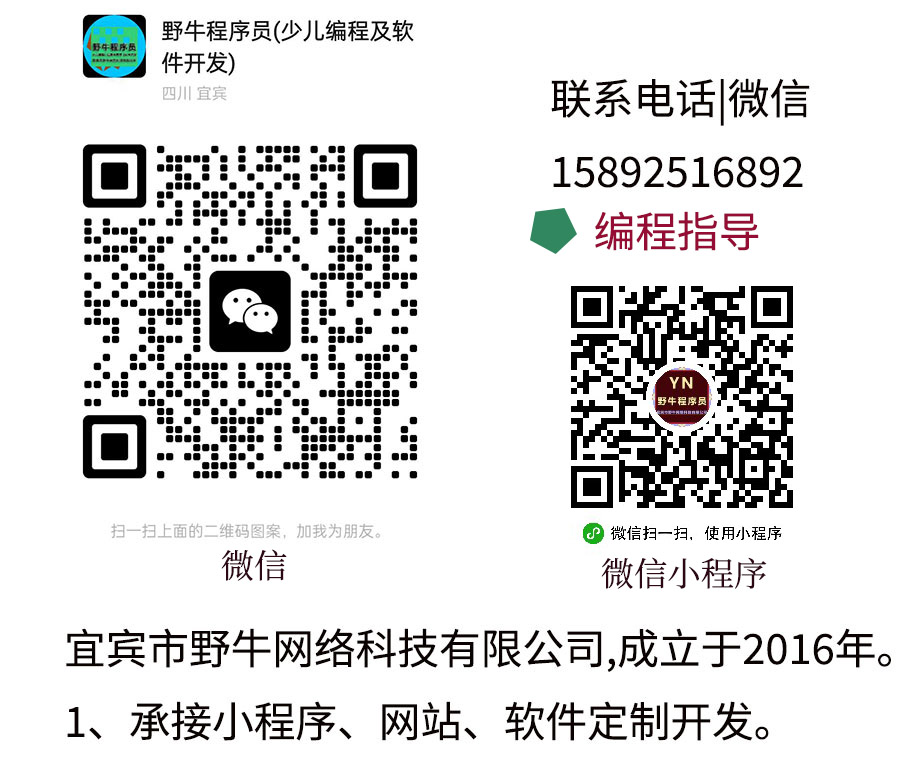