什么是最短路径优先算法?
作者:野牛程序员:2024-02-08 22:43:21 C++阅读 2153
最短路径优先算法是一种用于在加权图中查找从一个起始节点到所有其他节点的最短路径的算法。其中,"最短路径"通常是指路径上的边权重之和最小的路径。
最短路径优先算法的常见应用包括网络路由、地图导航和资源分配等领域。其中最著名的两种算法是Dijkstra算法和Bellman-Ford算法。
Dijkstra算法通过贪心策略逐步确定从起始节点到其他节点的最短路径。它维护一个优先队列来选择下一个要探索的节点,并在每次迭代中选择具有最小距离的节点进行探索,直到找到所有节点的最短路径。
Bellman-Ford算法是一种基于动态规划的算法,它可以处理带有负权边的图,并且能够检测负权环。该算法通过迭代更新节点之间的最短距离,直到收敛为止。
这些算法的选择取决于图的特性和应用需求。
以下是C++语言中实现Dijkstra算法的简单示例代码:
#include <iostream> #include <vector> #include <queue> #include <limits> using namespace std; #define INF numeric_limits<int>::max() // 边的结构体 struct Edge { int to; int weight; Edge(int t, int w) : to(t), weight(w) {} }; // 图的结构体 struct Graph { vector<vector<Edge>> adjacencyList; int size; Graph(int n) : size(n) { adjacencyList.resize(n); } // 添加边 void addEdge(int from, int to, int weight) { adjacencyList[from].emplace_back(to, weight); } }; // Dijkstra算法 vector<int> dijkstra(const Graph& graph, int start) { vector<int> dist(graph.size, INF); dist[start] = 0; priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq; pq.push({0, start}); while (!pq.empty()) { int u = pq.top().second; int d = pq.top().first; pq.pop(); if (d > dist[u]) continue; for (const Edge& e : graph.adjacencyList[u]) { int v = e.to; int w = e.weight; if (dist[u] + w < dist[v]) { dist[v] = dist[u] + w; pq.push({dist[v], v}); } } } return dist; } int main() { int n = 6; // 节点数量 Graph graph(n); // 添加边 graph.addEdge(0, 1, 5); graph.addEdge(0, 2, 3); graph.addEdge(1, 3, 6); graph.addEdge(1, 2, 2); graph.addEdge(2, 4, 4); graph.addEdge(2, 5, 2); graph.addEdge(2, 3, 7); graph.addEdge(3, 4, -1); graph.addEdge(4, 5, -2); int startNode = 0; // 起始节点 vector<int> shortestDistances = dijkstra(graph, startNode); cout << "Shortest distances from node " << startNode << ":\\n"; for (int i = 0; i < n; ++i) { cout << "Node " << i << ": " << shortestDistances[i] << endl; } return 0; }
这个代码示例包括一个简单的图数据结构和Dijkstra算法的实现。可以根据自己的需求修改图的结构和权重来测试算法的不同情况。
以下是C++语言中实现Bellman-Ford算法的简单示例代码:
#include <iostream> #include <vector> #include <limits> using namespace std; #define INF numeric_limits<int>::max() // 边的结构体 struct Edge { int from; int to; int weight; Edge(int f, int t, int w) : from(f), to(t), weight(w) {} }; // 图的结构体 struct Graph { vector<Edge> edges; int size; Graph(int n) : size(n) {} // 添加边 void addEdge(int from, int to, int weight) { edges.emplace_back(from, to, weight); } }; // Bellman-Ford算法 vector<int> bellmanFord(const Graph& graph, int start) { vector<int> dist(graph.size, INF); dist[start] = 0; // 进行|V| - 1轮松弛操作 for (int i = 0; i < graph.size - 1; ++i) { for (const Edge& edge : graph.edges) { if (dist[edge.from] != INF && dist[edge.from] + edge.weight < dist[edge.to]) { dist[edge.to] = dist[edge.from] + edge.weight; } } } // 检测负权环 for (int i = 0; i < graph.size - 1; ++i) { for (const Edge& edge : graph.edges) { if (dist[edge.from] != INF && dist[edge.from] + edge.weight < dist[edge.to]) { // 存在负权环 cout << "Graph contains negative weight cycle" << endl; return {}; } } } return dist; } int main() { int n = 5; // 节点数量 Graph graph(n); // 添加边 graph.addEdge(0, 1, -1); graph.addEdge(0, 2, 4); graph.addEdge(1, 2, 3); graph.addEdge(1, 3, 2); graph.addEdge(1, 4, 2); graph.addEdge(3, 2, 5); graph.addEdge(3, 1, 1); graph.addEdge(4, 3, -3); int startNode = 0; // 起始节点 vector<int> shortestDistances = bellmanFord(graph, startNode); cout << "Shortest distances from node " << startNode << ":\\n"; for (int i = 0; i < n; ++i) { cout << "Node " << i << ": " << shortestDistances[i] << endl; } return 0; }
这个代码示例包括一个简单的图数据结构和Bellman-Ford算法的实现。你可以根据自己的需求修改图的结构和权重来测试算法的不同情况。
野牛程序员教少儿编程与信息学奥赛-微信|电话:15892516892
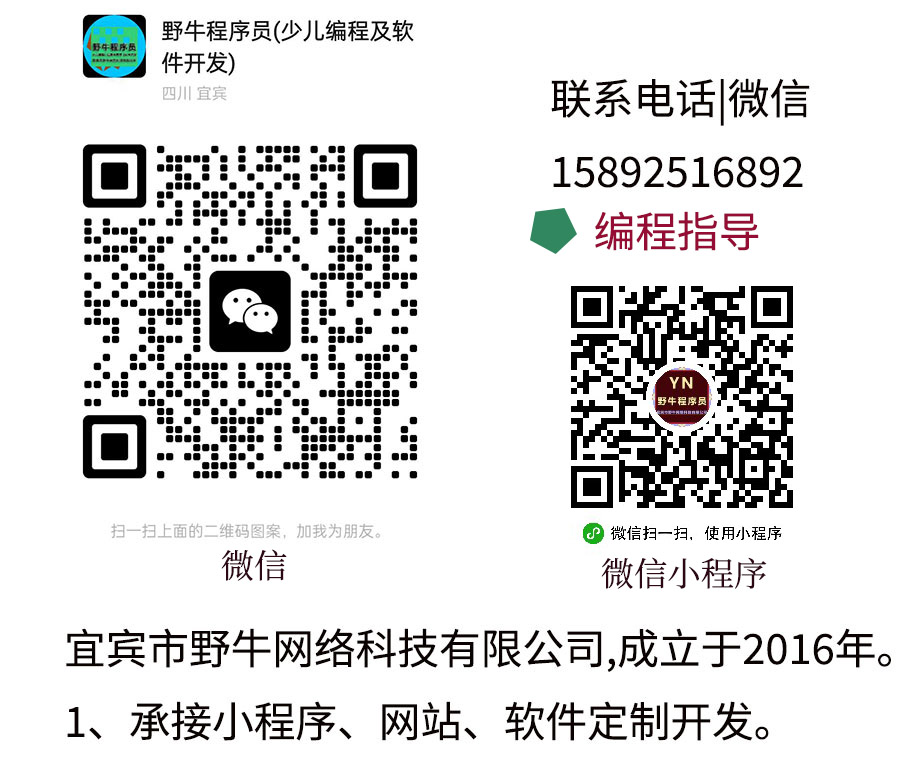